Dependency Inversion Principle
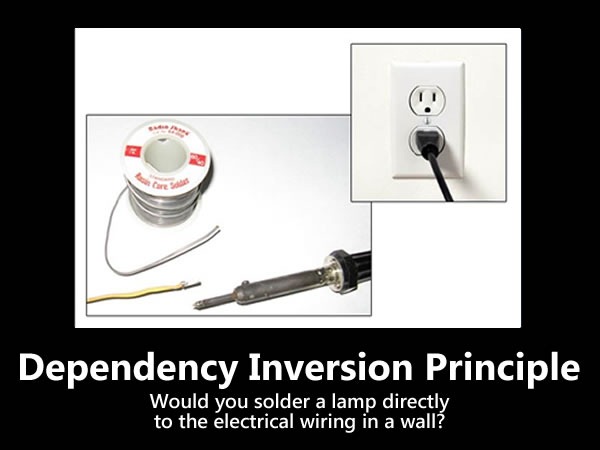
- ProductServiceBeforeDIP
namespace ConsoleApplication1
{
public class ProductServiceBeforeDIP
{
LinqToSqlProductRepository _productRepository;
public ProductServiceBeforeDIP()
{
_productRepository = new LinqToSqlProductRepository();
}
}
}
- ProductServiceAfterDIP
namespace ConsoleApplication1
{
class ProductServiceAfterDIP
{
IProductRepository _productRepository;
// using constructor to passing dependecy
public ProductServiceAfterDIP(IProductRepository productRepository)
{
_productRepository = productRepository;
}
public void GetAll()
{
// getting data
}
}
public interface IProductRepository
{
void GetAll();
}
}
- Demo
namespace ConsoleApplication1
{
class Program
{
static void Main(string[] args)
{
//1. using LinqToSql as data provider
ProductServiceAfterDIP productService = new ProductServiceAfterDIP(new LinqToSqlProductRepository());
productService.GetAll();
//2. using EF as data provider
productService = new ProductServiceAfterDIP(new EntityFrameWorkProductRepository());
productService.GetAll();
}
}
public class EntityFrameWorkProductRepository : IProductRepository
{
#region IProductRepository Members
public void GetAll()
{
// getting data with ef
}
#endregion
}
public class LinqToSqlProductRepository : IProductRepository
{
#region IProductRepository Members
public void GetAll()
{
// getting data with LinQtoSql
}
#endregion
}
}